STM32 Flash Memory Writing Guide Using HAL
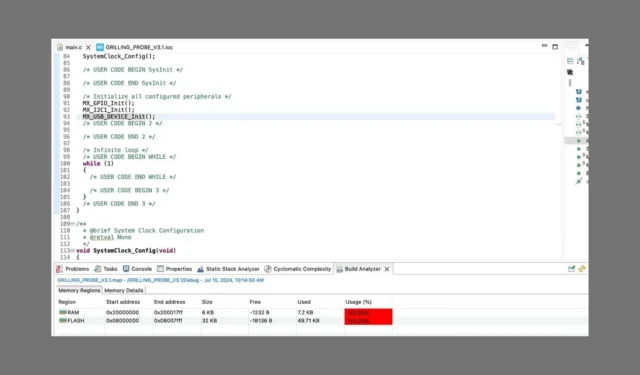
If you’ve chosen to utilize the STM32 flash memory with the Hardware Abstraction Layer (HAL), this guide will provide the essential steps you need for straightforward microcontroller programming. Setting up the Hardware Abstraction Layer (HAL) is quite manageable when following the examples outlined below.
How can I write to STM32 flash memory with HAL?
1. Get the flash memory ready for writing
- Incorporate necessary header files: Use
#include "stm32f4xx_hal.h"
. - Unlock the flash memory: You must unlock the flash memory prior to writing, by executing:
HAL_FLASH_Unlock();
. - Erase the Flash Memory: To write data, erase the specific flash memory sector you intend to use. This is accomplished with the HAL_FLASHEx_Erase function:
-
FLASH_EraseInitTypeDef EraseInitStruct; uint32_t SectorError; EraseInitStruct.TypeErase = FLASH_TYPEERASE_SECTORS; EraseInitStruct.Sector = FLASH_SECTOR_2; // Define the sector for erasure EraseInitStruct.NbSectors = 1; EraseInitStruct.VoltageRange = FLASH_VOLTAGE_RANGE_3; if (HAL_FLASHEx_Erase(&EraseInitStruct, &SectorError)! = HAL_OK) { // Error handling }
-
2. Writing data to flash memory
- Utilize the HAL_FLASH_Program function to input data into the flash memory. Data can be written in various forms: bytes, half-words, words, or double words.
-
uint32_t Address = 0x08008000; // Initial address in flash memory uint32_t Data = 0x12345678; // Information to be saved if (HAL_FLASH_Program(FLASH_TYPEPROGRAM_WORD, Address, Data)! = HAL_OK) { // Handle the error }
-
- Re-lock the flash memory: After completing the data write, it’s essential to lock the flash memory again to avoid any unintended overwrites:
HAL_FLASH_Lock();
.
Example code for STM32 flash memory with HAL
Below is a comprehensive example that amalgamates all the procedures:
#include "stm32f4xx_hal.h"
void Write_Flash(uint32_t Address, uint32_t Data) {
// Unlock the Flash
HAL_FLASH_Unlock();
// Erase the Flash sector
FLASH_EraseInitTypeDef EraseInitStruct;
uint32_t SectorError;
EraseInitStruct.TypeErase = FLASH_TYPEERASE_SECTORS;
EraseInitStruct.Sector = FLASH_SECTOR_2;
EraseInitStruct.NbSectors = 1;
EraseInitStruct.VoltageRange = FLASH_VOLTAGE_RANGE_3;
if (HAL_FLASHEx_Erase(&EraseInitStruct, &SectorError)! = HAL_OK) {
// Handle the error
}
// Program the Flash memory
if (HAL_FLASH_Program(FLASH_TYPEPROGRAM_WORD, Address, Data)! = HAL_OK) {
// Handle the error
}
// Lock the Flash
HAL_FLASH_Lock();
}
int main(void) {
HAL_Init();
Write_Flash(0x08008000, 0x12345678);
while (1) {
// Main loop
}
}
This illustration provides a complete demonstration of unlocking, erasing, writing to, and subsequently locking the flash memory of an STM32 microcontroller with HAL functionalities. We trust that this guide has been beneficial for your STM32 flash memory programming.
If you encounter any challenges, please refer to our tutorial on resolving the HAL INITIALIZATION FAILED error.
Should you have any queries or feedback, feel free to leave a comment below.
Leave a Reply