How to Fix Error Pipe Not Connected 233 (0xE9)
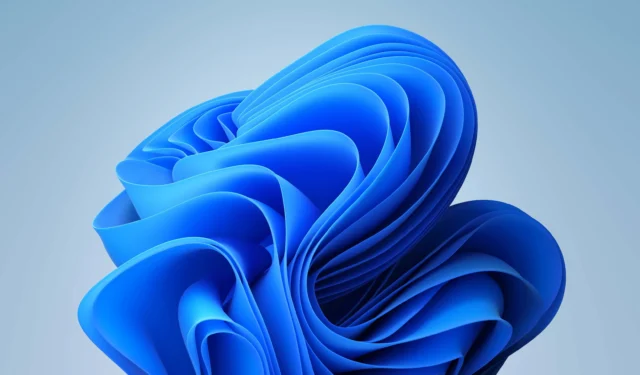
The ERROR_PIPE_NOT_CONNECTED is a common issue encountered by developers, typically accompanied by the message 233 (0xE9) stating that no process is connected to the pipe. If you run into this error, this article provides several solutions to resolve it effectively.
What steps can I take to resolve ERROR_PIPE_NOT_CONNECTED?
1. Modify your code
- Access your code files.
- Confirm that you have
PIPE_NOWAIT
defined in your code. - Implement it in your code as follows:
DWORD mode = PIPE_NOWAIT;SetNamedPipeHandleState(_callstackPipe,&mode,NULL,NULL);ConnectNamedPipe(_callstackPipe,NULL);mode = PIPE_WAIT;SetNamedPipeHandleState(_callstackPipe,&mode,NULL,NULL);
- Save the modifications.
2. Implement ConnectNamedPipe
- Review your code.
- If you encounter ERROR_PIPE_NOT_CONNECTED while using ReadFile, it indicates that the connection on the remote end has been lost.
- In this situation, consider employing ConnectNamedPipe without initially calling DisconnectNamedPipe.
3. Integrate a listener thread following ConnectNamedPipe()
- Open your code editor.
- Modify your implementation to include a listener thread, allowing for the next client connection as shown here:
Main Thread{ CreateListenerThread(); WaitForQuitEvent();}ListenerThread{ ConnectNamedPipe(); if (success) { CreateListenerThread(); if(PeekNamedPipe() detects a message) { ReadFile(); ProcessReceivedMessage(); // if a -quit signal is detected, trigger quit event } FileFlushBuffers(); DisconnectNamedPipe(); CloseHandle(); } else { // manage/report error }}
- Remember to save the changes.
4. Activate inheritance
- Access your code files.
- Incorporate the following line:
BOOL res = SetHandleInformation(hPipe, HANDLE_FLAG_INHERIT, HANDLE_FLAG_INHERIT);
- Save these updates.
This adjustment will enable inheritance for the handle, potentially resolving the issue.
Leave a Reply